티스토리 뷰
가장 먼저 시각화에 필요한 라이브러리를 불러옴.
# matplot
import matplotlib as mpl
import matplotlib.pyplot as plt
# seaborn
import seaborn as sns
# numpy와 pandas
import numpy as np
import pandas as pd
matplot 버전확인 방법
print(mpl.__version__)
가장 기본적인 사용법 (plt.plot())
# sample data
data_dict = {'x':[1, 2, 3, 4, 5], 'y':[6, 7, 8, 9, 10], 'y2':[1, 3, 5, 7, 9]}
plt.plot(data_dict['y']) # y값
plt.plot(data_dict['x'], data_dict['y']) # x값, y값
plt.plot('x', 'y', data=data_dict) # x값, y값 (위 코드와 동일한 의미)
# 하나의 plot 에 두 개의 선 그리기 (방법1)
plt.plot(data_dict['x'], data_dict['y'], data_dict['x'], data_dict['y2'])
# 하나의 plot 에 두 개의 선 그리기 (방법2)
plt.plot(data_dict['x'], data_dict['y'])
plt.plot(data_dict['x'], data_dict['y2'])
용어 정리
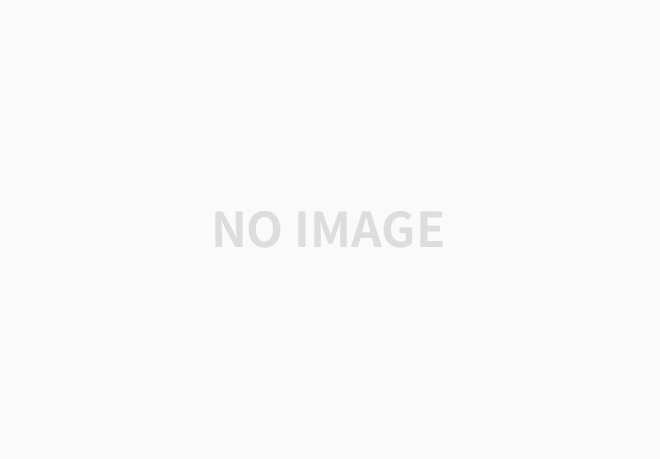
- figure: 도화지 / axes: 축 공간. 보통 plot으로 생각하는 그래프 / axis: 축. x축, y축의 제한 범위.
- 라벨: plt.xlabel(), plt.ylabel()
- 범례: plt.legend()
- 축: plt.xlim(xmin, xmax), plt.ylim(ymin, ymax), plt.axis([xmin, xmax, ymin, ymax]) -> ax[0].set_xlim(min, max)
- 그리드: plt.grid(True, axis='both')
- 눈금: plt.xticks(), plt.yticks() -> ax.set_xticks()
- 타이틀: plt.title() -> ax.set_title()
- gca: Get current Axes
matplot 공식 문서 정리
# A simple example
fig, ax = plt.subplots() # Create a figure containing a single axes.
ax.plot([1, 2, 3, 4], [1, 4, 2, 3]); # Plot some data on the axes.
- Figure: 전체 figure. Figure는 모든 자식 Axes(축 공간), Artists 그룹, 중첩된 하위 Figures까지 track(추적)함.
fig = plt.figure() # Axes(축 공간)이 없는 빈 Figure. 나중에 Axes를 수동으로 추가해줘야함.
fig = plt.figure(1, figsize=(20, 5))
- Axes: 데이터 플로팅을 위한 영역을 포함하는 Figure에 연결된 Artist. 일반적으로 눈금 및 눈금 레이블을 Axes의 데이터에 대한 척도를 제공. Axes 클래스와 그 멤버 함수는 OOP 인터페이스로 작업하기 위한 기본 진입점. 대부분의 플로팅 메서드가 정의되어 있음.
fig = plt.figure()
ax = fig.add_axes([0, 0, 1, 1]) # fig에 axes(축 공간)를 하나 추가. (left, bottom, width, height)
fig = plt.figure()
fig.add_subplot(111) # 서브플롯 arrangement(배열)의 일부로 fig에 axes(축 공간) 추가. (nrows, ncols, index)
ax = fig.add_subplot(111) # equivalent but more general
- plt.subplots: figure를 포함하여 서브플롯의 공통 레이아웃을 편리하게 만들 수 있는 유틸리티 래퍼.
plt.subplot(2,1,1) # (nrows, ncols, index)
plt.subplot(2,1,2)
- 가장 일반적인 방법: Figure와 Axes 동시 생성 (fig, ax = plt.subplots())
fig, ax = plt.subplots() # 하나의 Axes이 있는 Figure
fig, axs = plt.subplots(2, 2) # Axes의 2x2 그리드가 있는 Figure
# 가장 유용하게 쓸 수 있는 형식
fig, axes = plt.subplots(figsize=(20, 5), nrows=1, ncols=1, sharex=True, sharey=True) # fig와 서브플롯 세트를 만듦. x또는 y 축 간의 속성 공유 제어.
axes.plot() # Axes가 하나일 경우 이런식으로 Axes에 접근 (nrows=1, ncols=1)
axes[0].plot() # row, col 둘 중에 하나만 여러개일 경우 이런식으로 Axes에 접근 (nrows>1, ncols=1)
axes[0, 0].plot() # row, col 모두 여러개일 경우 이런식으로 Axes에 접근 (nrows>1, ncols>1)
- Axis: scale(크기)와 limit(제한)을 설정하고 눈금(Axis의 표시) 및 눈금 레이블(눈금에 레이블을 지정하는 문자열)을 생성. 눈금의 위치는 Locator 개체에 의해 결정되며 눈금 레이블 문자열은 Formatter에 의해 형식이 지정됨. 올바른 로케이터와 포맷터의 조합으로 눈금 위치와 레이블을 매우 세밀하게 제어할 수 있음.
- Artist: 기본적으로 Figure에 표시되는 모든 것은 아티스트(Figure, Axes 및 Axis 객체도 포함). 여기에는 Text 개체, Line2D 개체, 컬렉션 개체, Patch 개체 등이 포함. Figure가 렌더링되면 모든 아티스트가 캔버스에 그려짐. 대부분의 아티스트는 Axes(축)에 묶여 있음. 이러한 아티스트는 여러 Axes(축)에서 공유하거나 한 Axes(축)에서 다른 Axes(축)으로 이동할 수 없음.
- matplot 과 seaborn을 함께 쓰고 싶은 경우
sns.regplot(ax=axes) # axes-level function은 seaborn, matplotlib 섞어 쓸 수 있음.
- rcParams, savefig
# rcParams
plt.rcParams['figure.figsize'] = (6, 3)
plt.rcParams['font.size'] = 12
plt.rcParams['axes.grid'] = True
print(plt.style.available)
# savefig
plt.savefig('savefig_default.png')
'시각화' 카테고리의 다른 글
Streamlit 으로 모델 분류 모델 Inference 하기 (feat. SHAP) (1) | 2024.10.13 |
---|---|
Streamlit 알아보기 (2) (0) | 2024.05.25 |
Streamlit 알아보기 (1) (0) | 2024.05.25 |
mdates를 이용해 Matplotlib의 DateTime 눈금 빈도를 변경하는 방법 (0) | 2023.05.02 |
plotly (0) | 2023.01.04 |
- Total
- Today
- Yesterday
공지사항
최근에 올라온 글
글 보관함